This guide will delve into the most straightforward method for ensuring a background image stretches across the entire browser viewport. We’ll utilize the CSS `background-size` property to achieve this, without the need for JavaScript. [View Demo][Download Source from GitHub]
Currently, it’s quite trendy to have a full-background image on web pages.
Here are several websites featuring responsive full-background images: 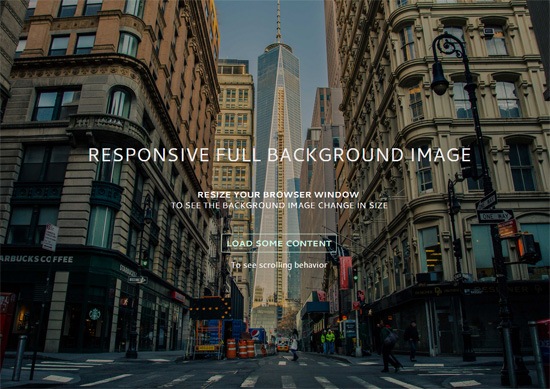, 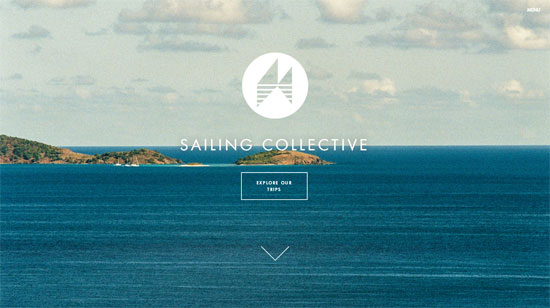, 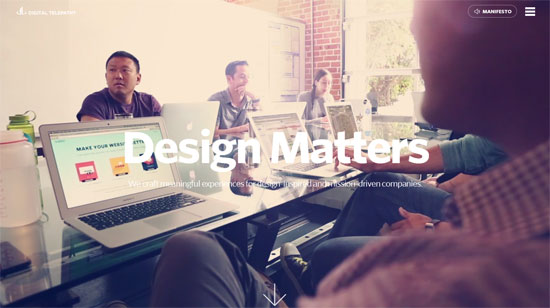, 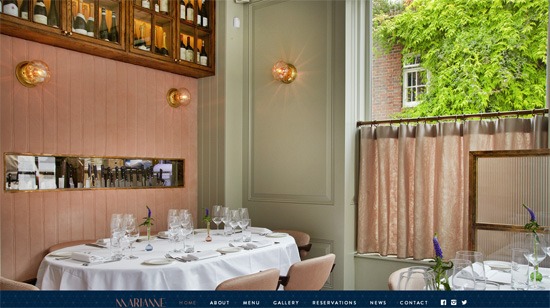. If you aim to replicate this effect in your upcoming web design project, you’ve come to the right place.
Here’s our approach:
Utilize the background-size property to cover the entire viewport
The `background-size` CSS property can be set to `cover`. This value instructs the browser to automatically and proportionally scale the background image’s width and height to ensure they are equal to or larger than the viewport’s width and height.
Implement a media query to serve a smaller background image for mobile devices
For improved page load speed on smaller screens, we’ll use a media query to serve a downscaled version of the background image. [Optional]
This technique functions without this step.
However, serving a smaller background image for mobile devices is a good idea. The image in the demo is approximately 5500x3600px. This size is suitable for most wide-screen monitors on the market today, but at the cost of serving a 1.7MB file.
A large file size for a background photo is never ideal, especially on mobile internet connections, where it can be particularly detrimental.
Additionally, the image dimensions are excessive for small-screen devices (we’ll explore this further in the tutorial).
Let’s walk through the process.
Here’s all you need for the markup:
<!doctype html> <html> <body> …Your content goes here… </body> </html>
We’ll assign the background image to the “ element, ensuring the image covers the entire browser viewport. This technique is also applicable to any block-level element (like a “ or a “). If the width and height of your block-level container are fluid, the background image will always scale to cover the container entirely.
We declare a style rule for the “ element as follows: body { /* Location of the image */ background-image: url(images/background-photo.jpg); /* Background image centered vertically and horizontally */ background-position: center center; /* Background image does not tile */ background-repeat: no-repeat; /* Background image fixed in the viewport */ background-attachment: fixed; /* Background image rescales based on container size */ background-size: cover; /* Background color displayed while image is loading */ background-color: #464646; }
The most crucial property-value pair to focus on is:
background-size: cover;
This is where the magic happens.
This property-value pair instructs the browser to scale the background image proportionally, ensuring its width and height are equal to or greater than the element’s width and height. (In our case, the “ element.)
However, there’s an issue with this property-value pair: If the background image is smaller than the “ element’s dimensions — which can occur on high-resolution screens or when there’s a lot of content on the page — the browser will programmatically upscale the image. As we all know, upscaling an image from its original dimensions can degrade image quality (i.e., pixelation occurs).
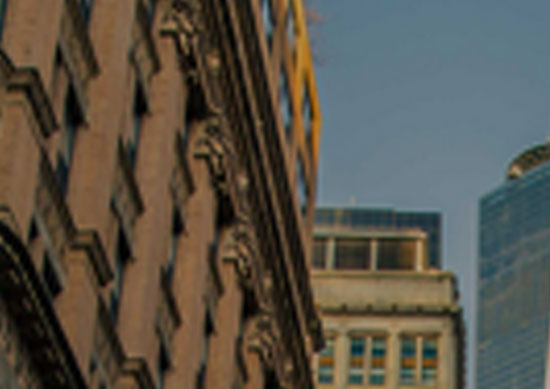 Keep this in mind when selecting your image. The demo uses a large 5500x3600px photo for larger screens, so we’ll encounter issues later. Let’s move on.
For the background image to remain centered in the viewport, we declare:
background-position: center center;
This sets the scaling axis at the center of the viewport. Next, we need to address the scenario where the content’s height exceeds the visible viewport’s height. When this occurs, a scrollbar will appear.
We want to ensure the background image remains stationary when the user scrolls down, preventing the image from running out or moving as the user scrolls (which can be very distracting). To achieve this, we set the `background-attachment` property to `fixed`.
background-attachment: fixed;
In the demo, I included a “load some content” feature so you can observe the scrolling behavior when `background-attachment` is set to `fixed`. One thing you could do is download the demo and experiment with the positional property values (e.g., `top` and `left`) to see how they affect page scrolling and the background image. The other property values are self-explanatory.
Shorthand CSS notation
I’ve written the background properties in full notation to make the CSS easier to understand.
The equivalent shorthand CSS notation for the above is: body { background: url(background-photo.jpg) center center cover no-repeat fixed; } All you need to do is change the `url()` value to point to the location of your background image, and you’re all set.
Optional: Media query for small screens
For small screens, I used Photoshop to resize the original background image proportionally to 768x505px and ran it through Smush.it to reduce the file size further, from 1741KB to 114KB. This results in a 93% reduction in file size.
Keep in mind that 114KB is still quite large for an aesthetic design component. For a 114KB payload, I would typically only subject users to it if the file had the potential to significantly improve UX, given the substantial mobile web performance trade-off. Here’s the media query: @media only screen and (max-width: 767px) { body { /* The file size of this background image is 93% smaller to improve page load speed on mobile internet connections */ background-image: url(images/background-photo-mobile-devices.jpg); } }
The downside of using the above media query is that if you resize your browser window from, for example, 1200px width down to 640px width (or vice versa), you will momentarily see a flicker while the smaller or larger background image loads. Additionally, because some small devices can render more pixels — for instance, the iPhone 5 with its retina display can render 1136x640px — the smaller background image may appear pixelated.
You can obtain the most current source code for this tutorial from GitHub. If I could offer one cautionary note about this technique, it would be this: Use it with care, as large files can significantly impact UX, particularly when the user is not on a fast or reliable internet connection.
This is also why you should set a good default background color so the user can read the content while the background image is loading. Optimizing your web images before deploying them is also a great practice; we have some articles to help with this:
Guide to Saving Images for the Web
Tools for Optimizing Your Images
A Crash Course Guide on JPEG
Advanced Image Optimization Tricks
Feel free to use the source code included in this tutorial without seeking permission; I’ve placed the tutorial’s code in the public domain under CC0 1.0 Universal. The source code in my GitHub repo is free of any copyright restrictions. You can use, sell, modify, and distribute the source code without asking for permission, providing attribution, or any other requirement.
(I don’t own the background image; it’s from Unsplash.)
Understanding the Elements of Responsive Web Design
8 Ways to Add a Responsive Navigation Menu on Your Site
A Comparison of Methods for Building Mobile-Optimized Websites